In this article, I will show how to create an identicon generator using Vue.js
in codepen. First of all, an identicon is a graphical image that is generated by text input. This is useful as a substitute for photo images for site users.
For example, when I type “Chris Nielsen Code Walk”, the identicon generator creates the image seen here:
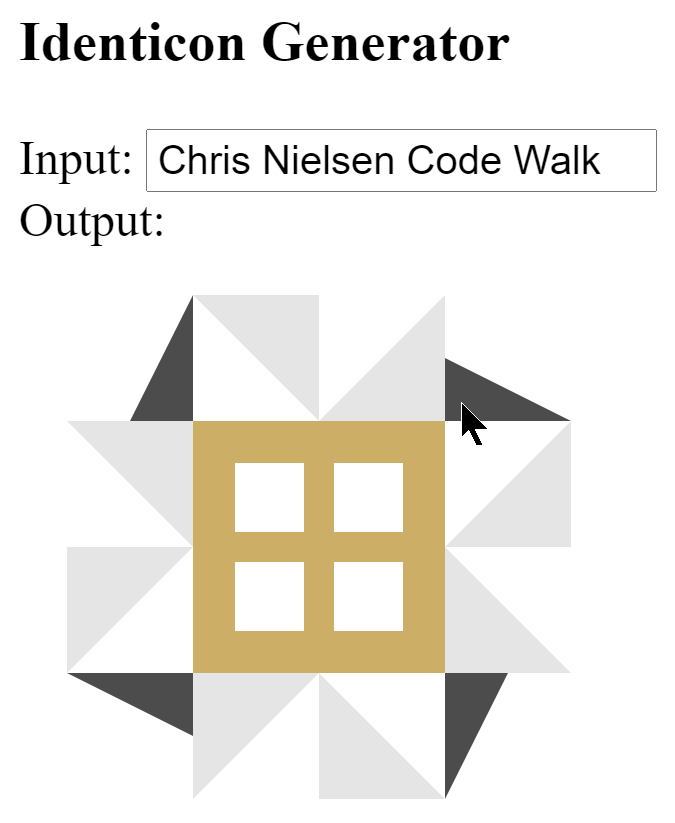
The identicon is generated via an external library called “jdenticon”. In codepen, we first need to go to the JS settings and pull in two libraries:
https://cdn.rawgit.com/dmester/jdenticon/5bbdf8cb/dist/jdenticon.min.js
and
https://cdnjs.cloudflare.com/ajax/libs/vue/2.6.11/vue.min.js
These can be found by clicking the settings icon next to JS and typing in “jdenticon” or “vue” and selecting one of the CDN results. For example:
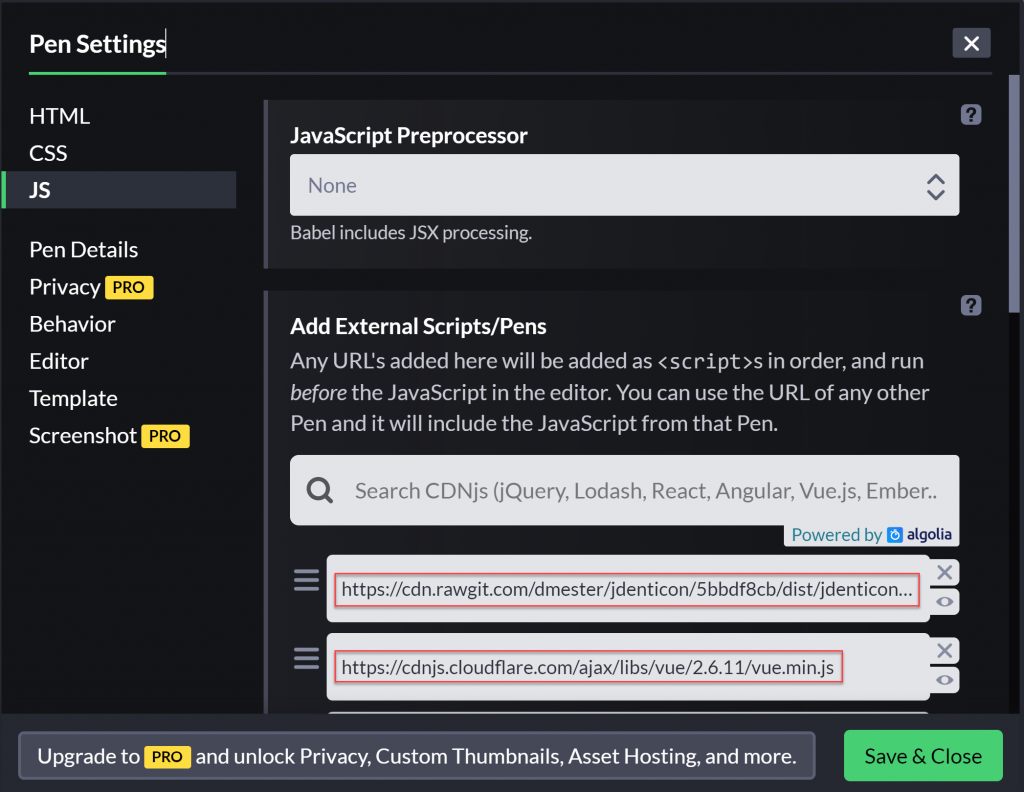
Starting with the HTML section in codepen, all we need for this Vue.js app is a single div with the id of the app. For example:
<div id="app"></div>
The rest of the code will be in the JavaScript section. First we need a new Vue constructor:
new Vue({ });
Then we need to tell Vue what div in the HTML to target. This can be done with an el: 'target'
. For example:
new Vue({ el: '#app', });
In the Vue constructor, we can set up our data object, methods, computed section, and template. For example:
new Vue({ el: '#app', data: { // key-value pairs textInput: '' }, computed: { // Turn our data into viewable values with these functions identicon: function() { return jdenticon.toSvg(this.textInput, 200); } }, methods: { // Use these functions to change data onInput: function(event) { // console.log(event.target.value); this.textInput = event.target.value; } }, template: ` <div> <h3>Identicon Generator</h3> <div> Input: <input v-on:input="onInput"/> <!-- The above line is a vue "directive" --> <!-- v-on = event handler --> <!-- The event is input --> <!-- onInput is the vue method that is called --> </div> <div> Output: <!-- {{ identicon }} --> <div v-html="identicon"></div> </div> </div> ` });
The complete app can be seen in my codepen:
See the Pen Vue Identicon Generator by Chris Nielsen (@Chris_Nielsen) on CodePen.dark