I was working on a video player app in Vue and forgot about the API_KEY that I was using from google for fetching video data from YouTube which I had listed as a const variable in my App.vue file. This ended up getting pushed up to my public github repo, exposing the key that I was issued. For example:
const API_KEY = AIzaSyBFpwlb38eH6BDR-8wgLOhOw9jhRXpN2G8; // Not a valid key
To fix this security issue so as not to allow unauthorized usage of my google API key, I did the following.
1. Created a new file in my project src directory called API_KEYS.txt
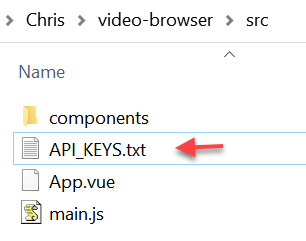
2. Pasted the API key there, on the top line
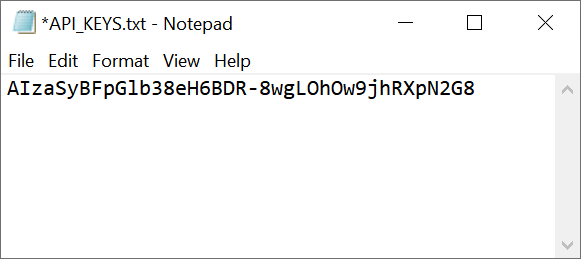
3. Added ‘API_KEYS.txt’ to my .gitignore
file so that this file won’t get pushed up to to github
4. In a cmd prompt, cd to the project directory and ran this command to install a module called raw-loader
:
npm install raw-loader --save-dev

5. After npm was finished installing the raw-loader module, I created a new file in the project root called vue.config.js
.
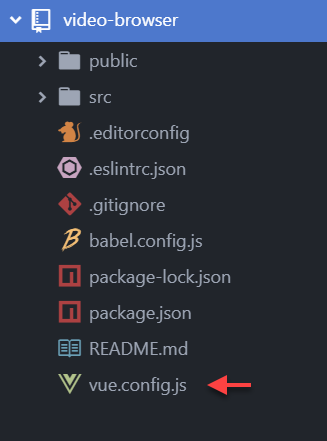
6. With this file created, I pasted the following text in it (to be used by npm and raw-loader):
module.exports = { chainWebpack: config => { config.module .rule('raw') .test(/\.txt$/) .use('raw-loader') .loader('raw-loader') .end() } }
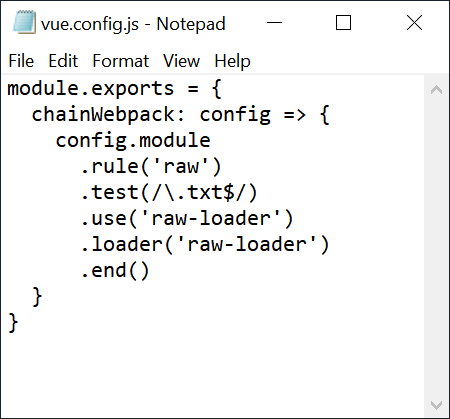
7. Now in the App.vue file, I added a new import statement to pull in the API key from APP_KEYS.txt and updated the API_KEY const variable to pull in that value so that the key is not visible in the code.
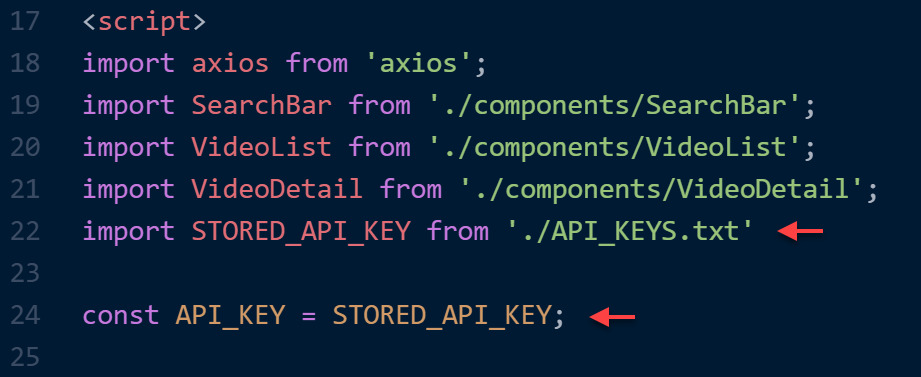
8. I then revoked the API key that had already been published on github and got a new API key from https://console.cloud.google.com/ by logging into my account and clicking the REGENERATE KEY button. I then pasted the newly regenerated key into API_KEYS.txt.
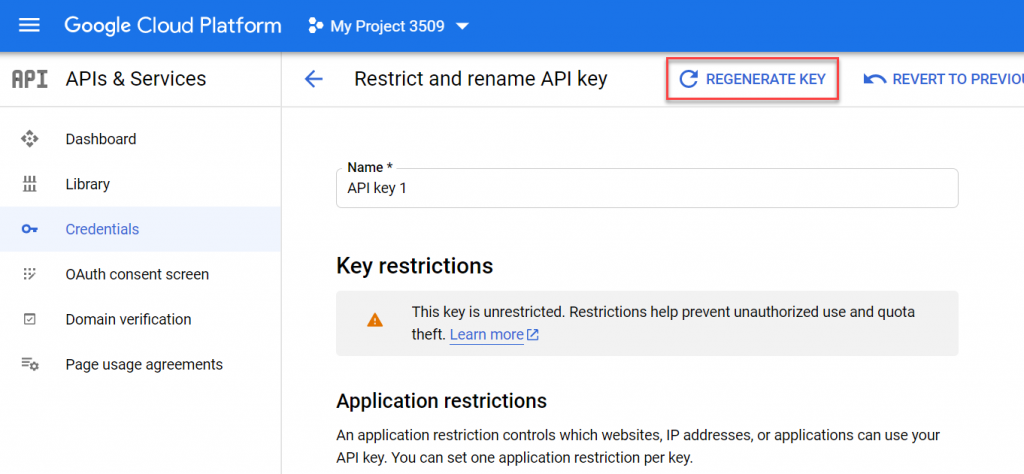
9. I then rebuilt the project in order to confirm that Vue was using the updated API_KEY and was still working.
10. Finally, I used git to push all of these changes up to the github repo. Now the old key is no longer valid and the new API key is not visible to the public.