The JavaScript ASCII Engine is a web tool I created to be able to quickly find ASCII values when a chart is not available. All you do is type in a single keyboard character in the input box and the tool will output the ASCII equivalent values in decimal, hex and binary.
I created this tool in the year 2000, and it has been featured on the JavaScript Source, as well as other free JavaScript source repositories. I checked a few years ago and found this tool being used on university websites around the world.
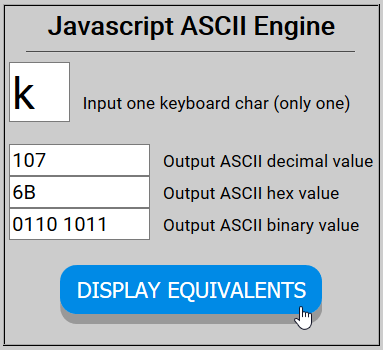
What is ASCII?
ASCII stands for American Standard Code for Information Interchange. It is a standard whereby symbols are represented by numbers in the computer. Every ASCII character can be represented by a number in the form of a decimal, hex, or even binary.
What is hex?
Hex is short for hexadecimal, which is a base 16 number system.
Why ??
It is useful for programmers to see these values when they are working on the bit level. That’s why the binary is included, because those eight binary digits are how the computer sees each character. One example of how this is useful is for encryption projects where the programmer is manipulating the character on the bit level.
Here is the JavaScript code that makes this tool work:
<SCRIPT LANGUAGE="JavaScript"> //Javascript Ascii Engine //Written by Christopher Nielsen //Jan 11, 2000 // Reset all fields to blank whenever page reloads function clearOnReload() { document.forms['asciiform'].reset(); } binary_numbers = new Array("0000", "0001", "0010", "0011", "0100", "0101", "0110", "0111", "1000", "1001", "1010", "1011", "1100", "1101", "1110", "1111"); function toAscii () { var symbols = " !\"#$%&'()*+,-./0123456789:;<=>?@" var loAZ = "abcdefghijklmnopqrstuvwxyz" symbols+= loAZ.toUpperCase() symbols+= "[\\]^_`" symbols+= loAZ symbols+= "{|}~" var loc = symbols.indexOf(document.asciiform.Input.value) if (loc > -1) { Ascii_Decimal = 32 + loc; return (32 + loc); } return(0) // If not in range 32-126 return ZERO } function Dec2Hex(Decimal) { var hexChars = "0123456789ABCDEF"; var a = Decimal % 16; var b = (Decimal - a)/16; hex = "" + hexChars.charAt(b) + hexChars.charAt(a); L = hexChars.charAt(a); H = hexChars.charAt(b); return hex; } function toBinary(High, Low) { var hiHex = "ABCDEF"; if(Low < 10 ){ LowNib = Low } else { LowNib = 10 + hiHex.indexOf(Low); } if(High < 10 ){ HighNib = High } else { HighNib = 10 + hiHex.indexOf(High); } eight_bits = binary_numbers[HighNib] + " " + binary_numbers[LowNib]; return eight_bits; } function getConversions(some_value) { document.asciiform.toDec.value=toAscii(); document.asciiform.toHex.value=Dec2Hex(toAscii()); document.asciiform.binary.value=toBinary(H, L); } </script>
The HTML features an onload call to clearOnReload() so that the text fields are empty every time the page is reloaded:
<body bgcolor="#cccccc" onload="clearOnReload()">
This app also has a feature such that every time the app is reloaded, the cursor moves to the input box. This is achieved by using autofocus="autofocus"
in the input tag.
The table for the app, which includes calls to the JavaScript functions, looks like this:
<TABLE BORDER=1 CELLSPACING=0 CELLPADDING=4 > <TR> <TD><center><FONT SIZE=+2><B>Javascript ASCII Engine</B></FONT></center><HR WIDTH=90%> <FORM NAME="asciiform" onsubmit="return false"> <INPUT SIZE="1" NAME="Input" style="font-size:30pt; width: 50px; height: 50px;" MAXLENGTH="1" autofocus="autofocus" onChange="getConversions(this.value)"> Input one keyboard char (only one) <BR><BR><INPUT SIZE="10" NAME="toDec" style="font-size:14pt; font-family: 'Roboto', sans-serif;" onFocus=this.blur()> Output ASCII decimal value <BR><INPUT SIZE="10" NAME="toHex" style="font-size:14pt; font-family: 'Roboto', sans-serif;" onFocus=this.blur()> Output ASCII hex value <BR><INPUT SIZE="10" NAME="binary" style="font-size:14pt; font-family: 'Roboto', sans-serif;" onFocus=this.blur()> Output ASCII binary value <BR><BR> <TABLE BORDER=0 width=100% > <TR> <TD align=center> <center> <INPUT TYPE="button" class="button" VALUE="DISPLAY EQUIVALENTS" onClick="document.asciiform.toDec.value=toAscii()"> </center> <BR> </TD> </TR> </TABLE> </TD> </TR> </TABLE>
As can be seen from the code, this app supports only the ASCII range that is available on most American keyboards. Decimal values 0-31 and 127 are non-keyboard characters and are therefore not supported. Here is what an ASCII chart looks like (minus the binary values):
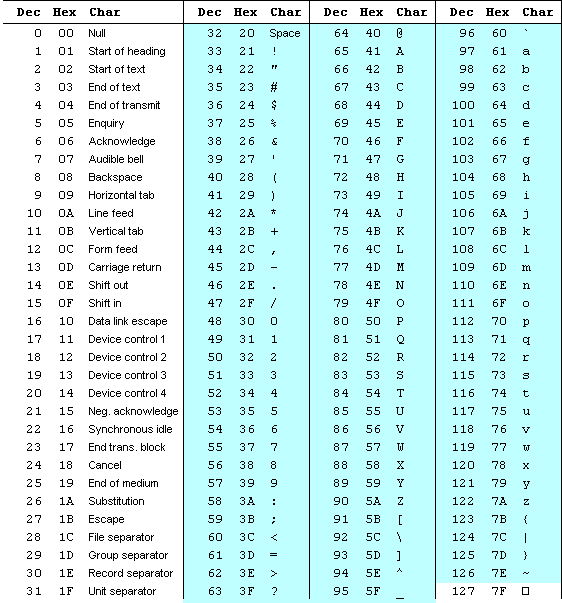
Here is the app, loaded in an iframe:
This can also be viewed directly on my website here:
https://bluegalaxy.info/js/ascii_engine.html