Recently I was working in a Piano template sandbox environment where I could edit HTML and CSS code to build a Piano template. This sandbox uses AngularJS, HTML, and CSS, but I wanted to see if it provided JavaScript and jQuery functionality so that I could enhance the template I was working on with some clickable animation.
I found out that JavaScripts would work in most templates by placing it in a div called “custom-script”. For example: <div custom-script>
Then to confirm whether or not JavaScript is enabled, I added a console.log()
to the top:
console.log("JS enabled")
Then I used this code to test whether jQuery is also installed. This not only confirms if jQuery is installed, it also tells me the version:
// Test to see if jQuery is enabled if (typeof(jQuery) == 'undefined') { console.log("jQuery not enabled") } else { console.log("jQuery enabled!", jQuery.fn.jquery) }
This is the code that tells me the actual jQuery version number: jQuery.fn.jquery
The complete code is:
<div custom-script> console.log("JS enabled") <!-- Test to see if jQuery is enabled --> if (typeof(jQuery) == 'undefined') { console.log("jQuery not enabled") } else { console.log("jQuery enabled!", jQuery.fn.jquery) } </div>
Which yields this in the console:
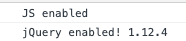
For more about piano see:
https://docs.piano.io/