Getting started with p5.js, this article follows the instructions found here:
https://p5js.org/get-started/
Where processing.py only allows interaction in the Processing code environment, p5.js allows for real time interactive experiences on the web. This article is similar to the previous article I wrote about getting started with Processing.py:
In the Processing IDE, choose the p5.js option at the right. For example:
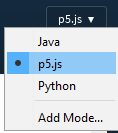
There are some syntactical differences between p5.js and regular Processing to make note of:
size()
has been replaced withcreateCanvas()
mousePressed
has been replaced withmouseIsPressed
Other differences are described here:
https://github.com/processing/p5.js/wiki/Processing-transition
The first project I completed with p5.js is the interactive circle_canvas project, which looks like this:
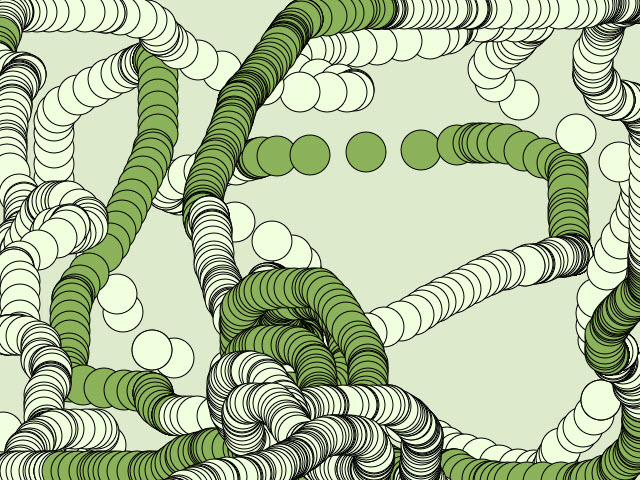
With p5.js projects, the Processing IDE provides two tabs, one for the code and one for the HTML needed to view the project on the web. The index.html file is created and updated automatically depending on name given to the project.
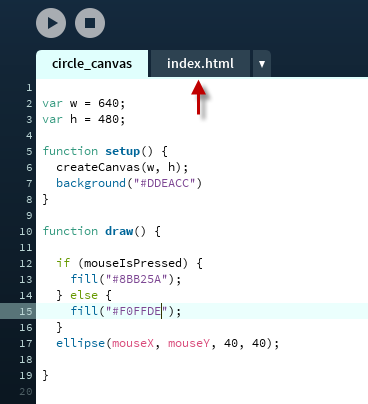
Here is the code for the circle_canvas project:
var w = 640; var h = 400; x = -50; // Initial position off screen y = -50; // Initial position off screen function setup() { createCanvas(w, h); background("#DDEACC") } function draw() { if (mouseIsPressed) { fill("#8BB25A"); } else { fill("#F0FFDE"); } // Use conditional to hide the initial circle off screen if (mouseX) { ellipse(mouseX, mouseY, 40, 40); } else { // hide off screen ellipse(x, y, 40, 40); } }
And here is the resulting index.html file that is automatically generated:
<html> <head> <meta charset="UTF-8"> <!-- PLEASE NO CHANGES BELOW THIS LINE (UNTIL I SAY SO) --> <script language="javascript" type="text/javascript" src="libraries/p5.js"></script> <script language="javascript" type="text/javascript" src="circle_canvas1.js"></script> <!-- OK, YOU CAN MAKE CHANGES BELOW THIS LINE AGAIN --> <!-- This line removes any default padding and style. You might only need one of these values set. --> <style> body {padding: 0; margin: 0;} </style> </head> <body> </body> </html>
Here is the interactive canvas for this project: