Once you have successfully written some node.js code and have viewed the results via command line, (see my previous article about this, linked below) the next logical step is to be able to write node.js code that can use HTML and be viewable in a web browser.
To view node.js output in a web browser, we can use the node.js module http
. This can be pulled into the script using a ‘require’. For example:
var http = require('http');
With the ‘http’ module pulled in via require, here is the rest of the setup needed to use a temporary server in node:
http.createServer(function (req, res) { res.writeHead(200, {'Content-Type': 'text/html'}); res.write("<h1>Hello World!</h1>\n\n"); res.end(); }).listen(8080);
The http module calls a function called createServer
. Notice the .listen(8080)
at the bottom. This indicates that we will be able to see the script output in our browser at localhost:8080.
Note: You can use any open port you want for this. For example .listen(3001)
would work also.
Some other things to notice about this setup:
- In res (short for response),
writeHead
, I used a Content-Type of ‘text/html’ - The
write
function is used for setting what you want output to the browser screen - There is a
res.end();
Here is what the complete code looks like so far:
var http = require('http'); http.createServer(function (req, res) { res.writeHead(200, {'Content-Type': 'text/html'}); res.write("<h1>Hello World!</h1>\n\n"); res.end(); }).listen(8080);
With all of this setup complete, I saved the document as ‘hello.js’.
Now if we go straight to this web location in our browser, we will see some kind of “unable to connect” message, because we haven’t yet initiated the server via the command line. For example, in Firefox I saw this :
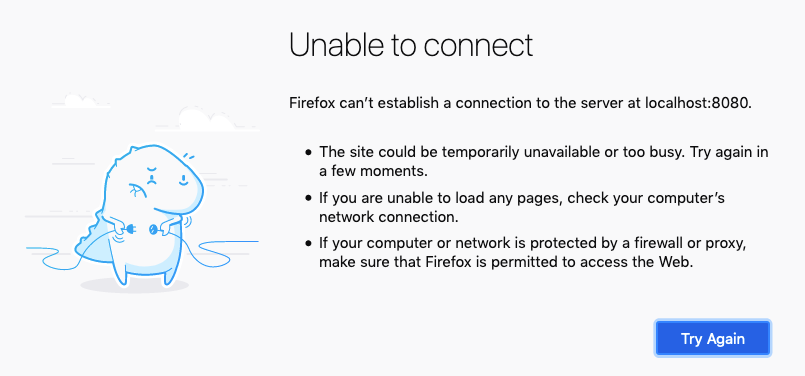
So in order to launch the temporary server to see the output in our browser, we must run the node.js script via the command line. For example:

Now when I refresh the browser, I see the expected output:
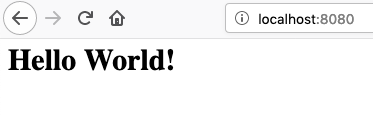
You may notice that the command line is stuck on the line after ‘node hello.js’. In order to get control back in the command window we can type CTRL+c
on Windows or CMD+c
on Mac.
Note: The anonymous function call in the code sample shown above is actually a callback function! Another way to look at what is going on is to give the callback function a name (“handleRequest”) and separate it out from the http.createServer() function call. For example:
function handleRequest(req, res) { res.writeHead(200); res.end("hello world\n"); } https.createServer(options, handleRequest).listen(8080);
For more information about the http.createServer()
function, see:
https://nodejs.org/api/http.html#http_http_createserver_options_requestlistener
For more information about the res.writeHead()
function, see:
https://nodejs.org/api/http.html#http_response_writehead_statuscode_statusmessage_headers
For more information about the res.end()
function, see:
https://nodejs.org/api/http.html#http_response_end_data_encoding_callback