Let’s say you’re working on an API that will create JSON data and you want to store that data in an S3 bucket for retrieval by a separate Lambda script.
Note: For this code example, I am using node.js as my runtime language in my AWS Lambda.
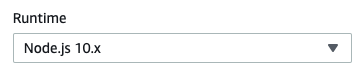
Let’s say the JSON data has been created and now it is time to write that data to the S3 bucket.
The first step is to set up the following in the require
portion of your node.js code:
const AWS = require('aws-sdk'); const S3 = new AWS.S3({region: process.env.AWS_REGION, apiVersion: '2012-10-17'});
Then add this function to the Lambda:
function putObjectToS3(data, nameOfFile) { //Write to file var s3 = new AWS.S3(); var params = { Bucket : "name_of_s3_bucket", Key : nameOfFile + ".json", Body : data } s3.putObject(params, function(err, data) { if (err) console.log(err, err.stack); // an error occurred else console.log("Put to s3 should have worked: " + data); // successful response }); }
Of course before this is called, you will need to set up the S3 bucket with proper read/write permissions. Then it can be called like this:
// stringify the consumable data that was created var jsonOutput = JSON.stringify(consumableData); // output the data to confirm it is correct console.log(jsonOutput); // call the function to write the data to the S3 bucket putObjectToS3(jsonOutput, "myData")
Then when you inspect the S3 bucket, you should see the file there:
